Form Builder - Layout
Section
Overview
You may want to separate your fields into sections, each with a heading and description. To do this, you can use a section component:
use Filament\Forms\Components\Section; Section::make('Rate limiting') ->description('Prevent abuse by limiting the number of requests per period') ->schema([ // ... ])

You can also use a section without a header, which just wraps the components in a simple card:
use Filament\Forms\Components\Section; Section::make() ->schema([ // ... ])

Adding actions to the section's header or footer
Sections can have actions in their header or footer.
Adding actions to the section's header
You may add actions to the section's header using the headerActions()
method:
use Filament\Forms\Components\Actions\Action;use Filament\Forms\Components\Section; Section::make('Rate limiting') ->headerActions([ Action::make('test') ->action(function () { // ... }), ]) ->schema([ // ... ])
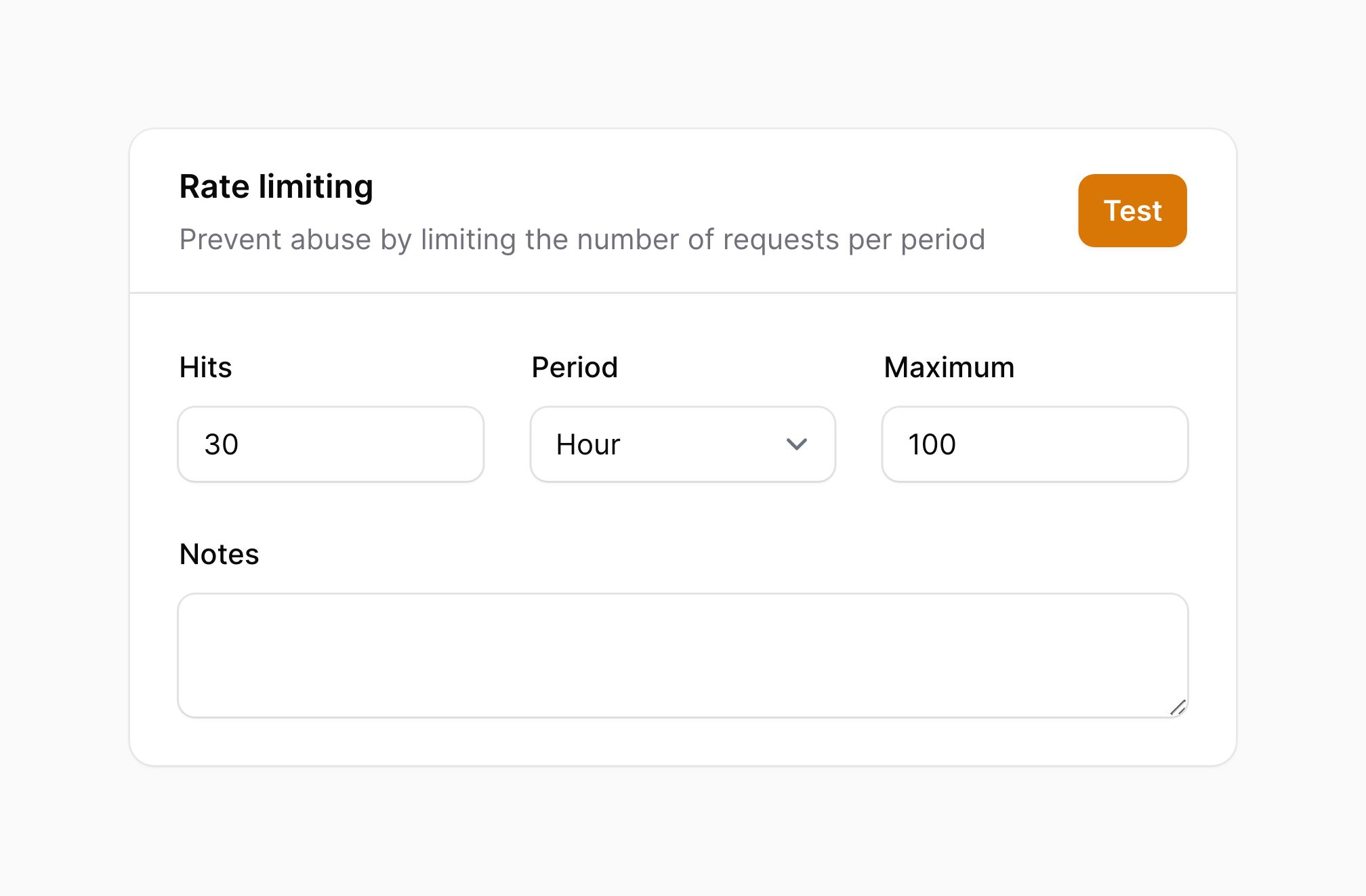
Adding actions to the section's footer
In addition to header actions, you may add actions to the section's footer using the footerActions()
method:
use Filament\Forms\Components\Actions\Action;use Filament\Forms\Components\Section; Section::make('Rate limiting') ->schema([ // ... ]) ->footerActions([ Action::make('test') ->action(function () { // ... }), ])

Aligning section footer actions
Footer actions are aligned to the inline start by default. You may customize the alignment using the footerActionsAlignment()
method:
use Filament\Forms\Components\Actions\Action;use Filament\Forms\Components\Section;use Filament\Support\Enums\Alignment; Section::make('Rate limiting') ->schema([ // ... ]) ->footerActions([ Action::make('test') ->action(function () { // ... }), ]) ->footerActionsAlignment(Alignment::End)
Adding actions to a section without heading
If your section does not have a heading, Filament has no way of locating the action inside it. In this case, you must pass a unique id()
to the section:
use Filament\Forms\Components\Section; Section::make() ->id('rateLimitingSection') ->headerActions([ // ... ]) ->schema([ // ... ])
Adding an icon to the section's header
You may add an icon to the section's header using the icon()
method:
use Filament\Forms\Components\Section; Section::make('Cart') ->description('The items you have selected for purchase') ->icon('heroicon-m-shopping-bag') ->schema([ // ... ])

Positioning the heading and description aside
You may use the aside()
to align heading & description on the left, and the form components inside a card on the right:
use Filament\Forms\Components\Section; Section::make('Rate limiting') ->description('Prevent abuse by limiting the number of requests per period') ->aside() ->schema([ // ... ])

Collapsing sections
Sections may be collapsible()
to optionally hide content in long forms:
use Filament\Forms\Components\Section; Section::make('Cart') ->description('The items you have selected for purchase') ->schema([ // ... ]) ->collapsible()
Your sections may be collapsed()
by default:
use Filament\Forms\Components\Section; Section::make('Cart') ->description('The items you have selected for purchase') ->schema([ // ... ]) ->collapsed()

Persisting collapsed sections
You can persist whether a section is collapsed in local storage using the persistCollapsed()
method, so it will remain collapsed when the user refreshes the page:
use Filament\Infolists\Components\Section; Section::make('Cart') ->description('The items you have selected for purchase') ->schema([ // ... ]) ->collapsible() ->persistCollapsed()
To persist the collapse state, the local storage needs a unique ID to store the state. This ID is generated based on the heading of the section. If your section does not have a heading, or if you have multiple sections with the same heading that you do not want to collapse together, you can manually specify the id()
of that section to prevent an ID conflict:
use Filament\Infolists\Components\Section; Section::make('Cart') ->description('The items you have selected for purchase') ->schema([ // ... ]) ->collapsible() ->persistCollapsed() ->id('order-cart')
Compact section styling
When nesting sections, you can use a more compact styling:
use Filament\Forms\Components\Section; Section::make('Rate limiting') ->description('Prevent abuse by limiting the number of requests per period') ->schema([ // ... ]) ->compact()

Using grid columns within a section
You may use the columns()
method to easily create a grid within the section:
use Filament\Forms\Components\Section; Section::make('Heading') ->schema([ // ... ]) ->columns(2)
Edit on GitHubStill need help? Join our Discord community or open a GitHub discussion